Midterm Project
The final project of this course is to build collision detection system.
In this midterm project, we will do the feature tracking part (test various detectors/descriptors combination).
This project is divided into 4 parts:
- Loading images, setting up data structures, and putting everything into a ring buffer to optimize the memory load.
- Integrate several keypoint detectors (Harris, FAST, BRISK, SIFT) and compare them to each other with regard to the number of keypoints and speed.
- Descriptor extraction and matching using Brute Force and FLANN approach.
- Test various algorithms and different combinations, and compare them with regard to some performance measures.
Part 1: The Data Buffer
Task MP.1
Your first task is to set up the loading procedure for the images, which is currently not optimal. In the student version of the code, we push all images into a vector inside a for-loop and with every new image, the data structure grows. Now imagine you want to process a large image sequence with several thousand images and Lidar point clouds over night - in the current implementation this would push the memory of your computer to its limit and eventually slow down the entire program. So in order to prevent this, we only want to hold a certain number of images in memory so that when a new one arrives, the oldest one is deleted from one end of the vector and the new one is added to the other end. The following figure illustrates the principle.
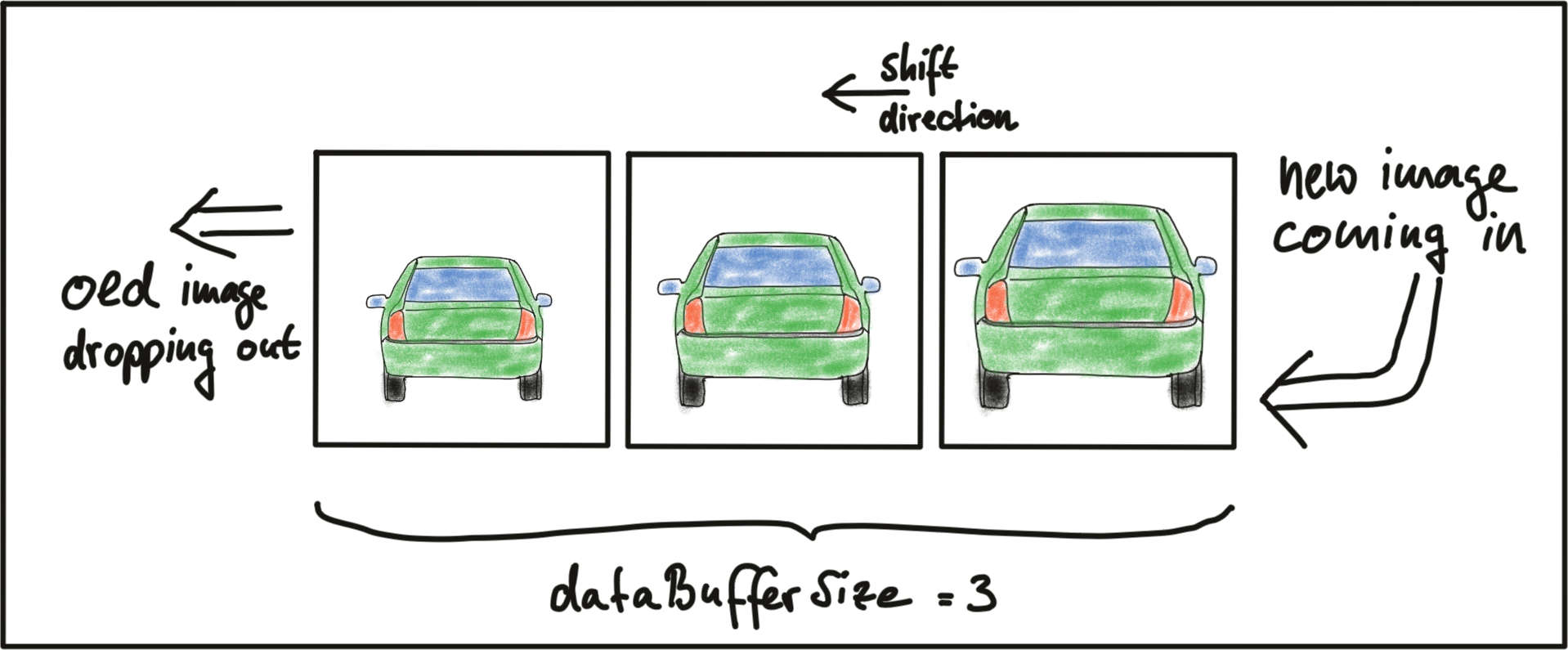
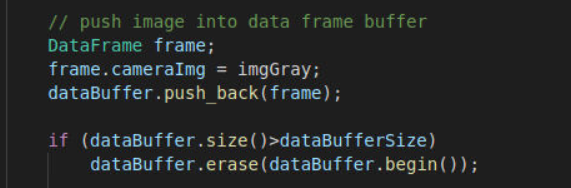
Part 2: Keypoint Detection
TASK MP.2
Your second task is to focus on keypoint detection. In the student version of the code you will find an existing implementation of the Shi-Tomasi detector. Please implement a selection of alternative detectors, which are HARRIS, FAST, BRISK, ORB, AKAZE, and SIFT. The following figure shows keypoints detected with the SIFT method.
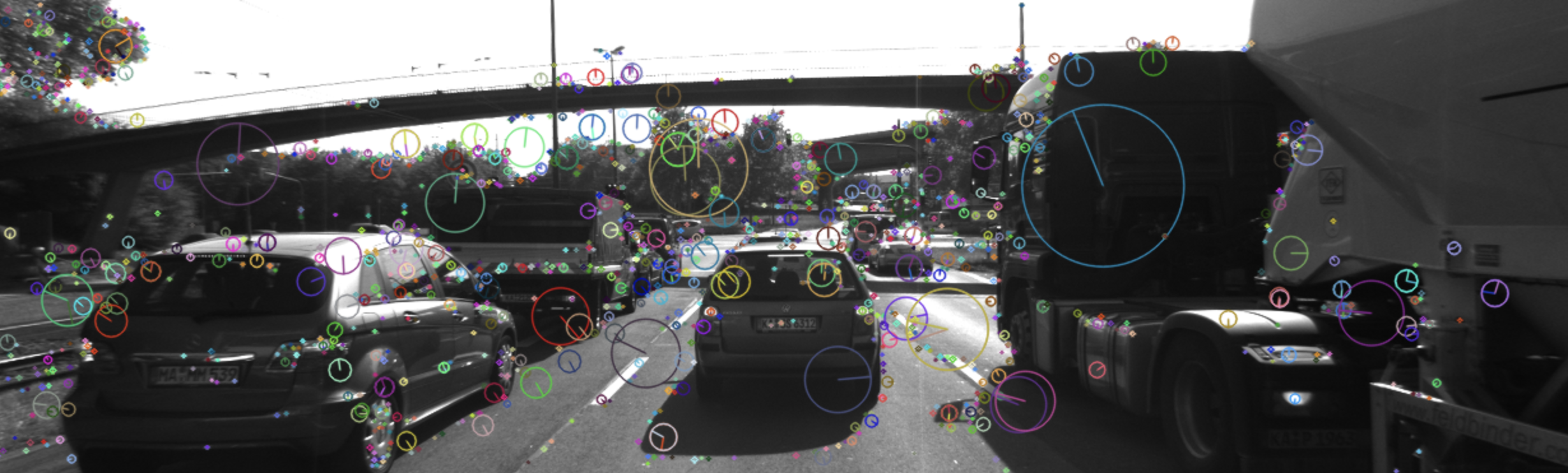
The Harris detector is already familiar to you from a previous exercise and the other methods are available in the OpenCV. Also, please adjust the project code in a way that each detector can be selected by setting the string 'detectorType' to the respective name. Please note that all detectors should be added to the file 'matching2D_student.cpp'. Take a look at the header file 'matching2D.hpp' for details on the call parameters for the functions you need to implement.